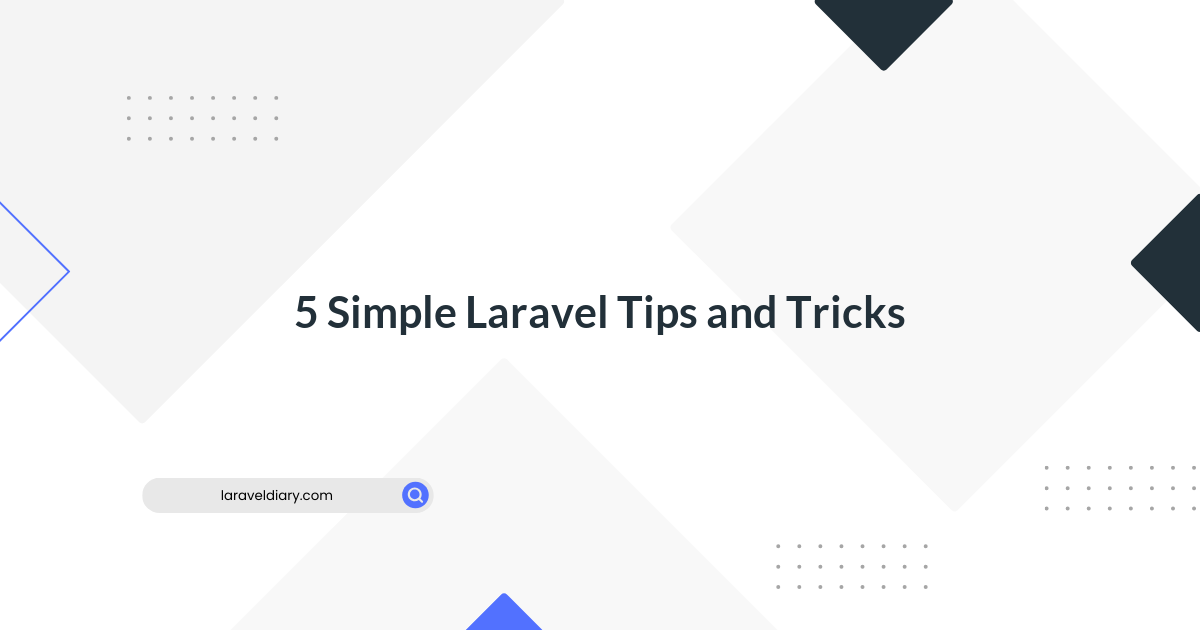
Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries (Series-6)
Table of Contents
- Scenario 26: Utilizing replicate for Cloning Models
- Scenario 27: Smart Relationship Refreshing with refresh
- Scenario 28: Avoid Overriding Primary Keys Unintentionally
- Scenario 29: Tactical Use of whereExists and whereNotExists
- Scenario 30: Restricting Relations with Global Scopes
- Series Conclusion: Mastering Eloquent for Fulgent Web Applications
- Previous Part of this Series
Continuing on from where we left off, let's expand your arsenal of Eloquent maneuvers with five additional scenarios that demonstrate the ORM's agility and power.
replicate
for Cloning Models
Scenario 26: Utilizing Good Practice: Make an exact copy of a model, except for its primary key, using the replicate
method.
$originalPost = App\\Models\\Post::find(1);
$clonedPost = $originalPost->replicate();
$clonedPost->save(); // Save the cloned model as a new record
Bad Practice: Manually setting attributes to create a new, similar record, which is error-prone.
$originalPost = App\\Models\\Post::find(1);
$clonedPost = new App\\Models\\Post($originalPost->toArray());
$clonedPost->id = null; // Remember to exclude the primary key
$clonedPost->save();
refresh
Scenario 27: Smart Relationship Refreshing with Good Practice: Update the parent model and all of its relationships using the refresh
method.
$post = App\\Models\\Post::with('comments')->find(1);
// ...after some updates to the comments
$post->refresh(); // The $post model and its 'comments' relationship are reloaded from the database
Bad Practice: Manually reloading the model and relations which could lead to inconsistencies.
$post = App\\Models\\Post::with('comments')->find(1);
// ...after some updates to the comments
$post->load('comments'); // Only the 'comments' relationship is refreshed, the parent $post model is untouched
Scenario 28: Avoid Overriding Primary Keys Unintentionally
Good Practice: Ensure mass assignment doesn't inadvertently overwrite primary key values by setting the $guarded
property appropriately.
class Post extends Model
{
protected $guarded = ['id']; // Guard the 'id' to prevent overwriting
}
Bad Practice: A lack of protection that allows mass assignments to modify primary keys.
class Post extends Model
{
// Without the 'id' guarded, a mass assignment could override it
protected $fillable = ['title', 'content']; // 'id' is not guarded
}
whereExists
and whereNotExists
Scenario 29: Tactical Use of Good Practice: Use whereExists
for queries that only need to check the presence of a related record.
$usersWithPosts = App\\Models\\User::whereExists(function ($query) {
$query->select(DB::raw(1))
->from('posts')
->whereRaw('posts.user_id = users.id');
})->get();
Bad Practice: An inefficient way of checking for related records' existence.
$usersWithPosts = App\\Models\\User::all()->filter(function ($user) {
return $user->posts()->exists();
});
Scenario 30: Restricting Relations with Global Scopes
Good Practice: Apply a global scope to a relation to enforce a constraint every time that relation is queried.
class ActiveCommentScope implements \\Illuminate\\Database\\Eloquent\\Scope
{
public function apply(Builder $builder, Model $model)
{
$builder->where('active', 1);
}
}
// Usage in the related model
class Post extends Model
{
protected static function booted()
{
static::addGlobalScope(new ActiveCommentScope);
}
public function comments()
{
return $this->hasMany(Comment::class);
}
}
// Every time you retrieve comments from a post, they will be active comments.
Bad Practice: Manually applying the same constraints repeatedly.
$post = App\\Models\\Post::find(1);
$comments = $post->comments()->where('active', 1)->get(); // Constraint is manually applied
Series Conclusion: Mastering Eloquent for Fulgent Web Applications
As we conclude this six-part series on advanced Eloquent techniques, it's evident that the true mastery of Eloquent lies in a developer’s ability to discern when and how to apply its plethora of utilities. Each scenario we've covered adds a layer of sophistication to your understanding of Eloquent, allowing you to craft eloquent queries with confidence and finesse.
From optimizing common queries across the application lifecycle to making astute use of the ORM's nuanced capabilities, these 30 scenarios are designed to inspire a deeper, more intuitive relationship with Laravel's Eloquent ORM. Not only can employing these advanced patterns optimize performance and maintainability, but it also underpins a philosophy of writing code that is both expressive and efficient — a testament to the thoughtfulness ingrained in Laravel itself.
Whether you're manipulating large datasets, enforcing business logic constraints, or streamlining database interactions, Eloquent's toolkit can accommodate–and the more proficient you are with these features, the more resplendent your applications will become.
Laravel, with Eloquent as its ORM, remains a framework that continues to astonish with its elegance, and the advanced tricks we've discovered are but a glimpse into what is achievable. Keep exploring, keep discovering, and most importantly, keep enjoying the art of crafting exceptional web applications with Laravel.
May your queries always return true, your relationships be eager, and your models perfectly cast. Laravel's Eloquent ORM stands ready to be your ally in the ceaseless journey of web development mastery.
Previous Part of this Series
Comments (0)
What are your thoughts on "Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries (Series-6)"?
You need to create an account to comment on this post.
Related articles
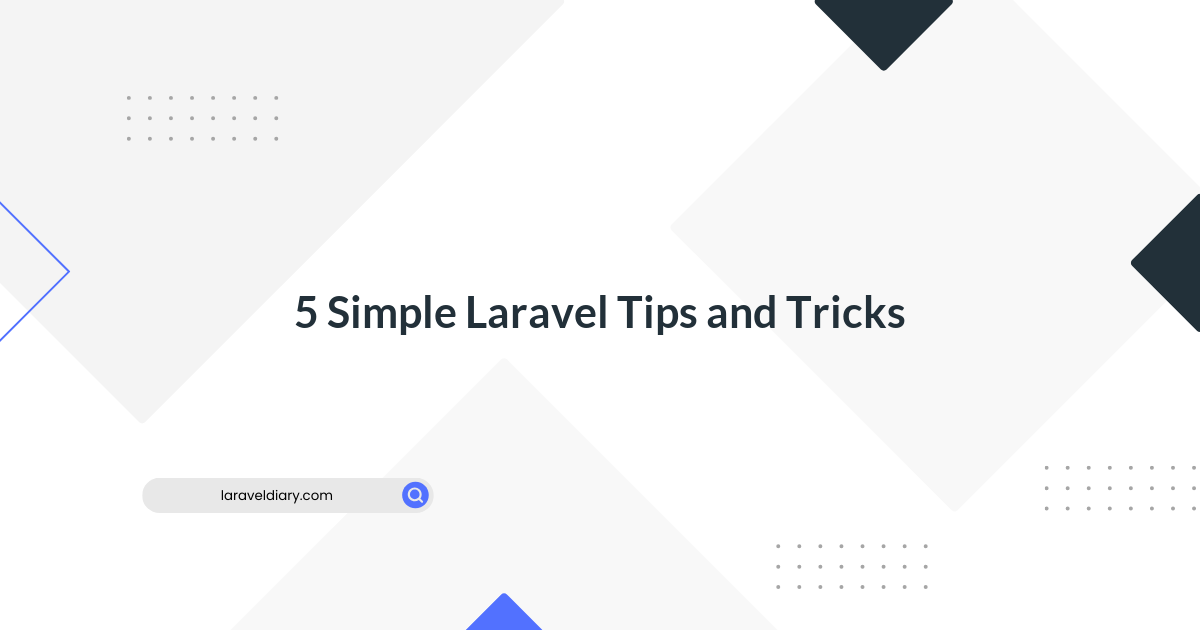