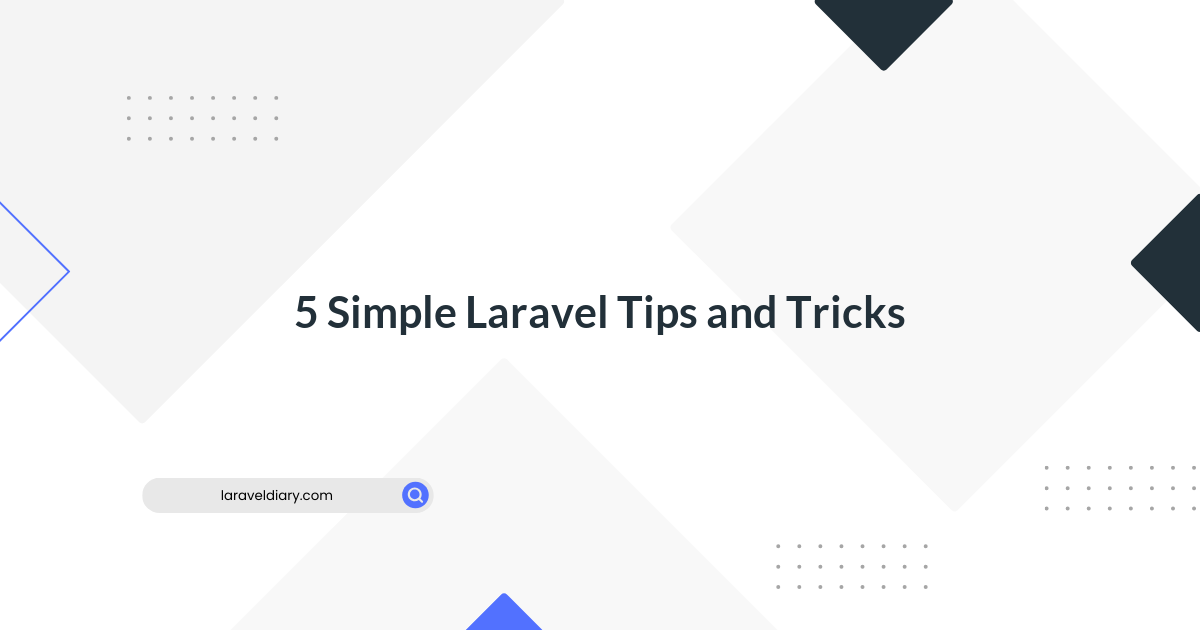
Navigating Timezones in Laravel: Storing and Managing Time with Precision
Table of Contents
- The Lay of the Land: Timezone Basics with PHP DateTime
- Setting the Stage with Laravel's now()
- Employing Carbon for Timezone Alchemy
- Integrating with Luxon.js on the Frontend
- Ensuring Time Integrity Across Database Types
- Conclusion: Mastering Timezone Management in Laravel
Imagine you're sculpting an international event planning application in Laravel, a stage where attendees, speakers, and vendors from across the globe gravitate. In such a global village, managing timezones is not just essential—it's the gravitational pull that keeps every satellite in orbit. The challenge, however, is ensuring that every timestamp stored in your database reflects the correct local time, and your application's frontend can interpret it without a hitch. Thankfully, Laravel, coupled with its Carbon package and regular PHP DateTime functions, offers seamless timezone management. Here's how you can harness these tools to ensure your application ticks right, every time, everywhere.
The Lay of the Land: Timezone Basics with PHP DateTime
Before we leap into Laravel and Carbon, let's ground ourselves with PHP's native DateTime class:
$dateTime = new DateTime('now', new DateTimeZone('Europe/London'));
echo $dateTime->format('Y-m-d H:i:s'); // Outputs the current time in London
While PHP DateTime gets the job right, it's not quite enough. We crave the syntactic sugar that Laravel offers. But understanding this basic operation is key as it's the foundation upon which Laravel builds.
Setting the Stage with Laravel's now()
In our event app, let's say we need to timestamp when a new event is created. Laravel provides the now() helper, a wrapper around Carbon for this very purpose:
use Illuminate\Support\Facades\DB;
DB::table('events')->insert([
'name' => 'Global Laravel Meetup',
'start_time' => now() // Inserts the current time based on the app's timezone
]);
now()
is context-aware, respecting the timezone specified in your config/app.php
. But the world does not revolve around one timezone—so how do we cater to our global audience?
Employing Carbon for Timezone Alchemy
Enter Carbon, the robust library that extends PHP's DateTime and comes pre-installed with Laravel. It's like DateTime on steroids, bringing functionality and ease of use that PHP's native offerings can only dream of.
Adjusting Timezones on the Fly
Using Carbon
, we can manipulate timezones effortlessly. Let's provide our users with times that resonate with their locales:
use Carbon\Carbon;
$eventTime = Carbon::createFromFormat('Y-m-d H:i', '2023-10-30 19:00', 'UTC');
$userTimezone = 'America/New_York';
$localEventTime = $eventTime->setTimezone($userTimezone);
echo $localEventTime; // Outputs the event time in 'America/New_York' timezone
This flexibility allows our application to cater local times to users, irrespective of where they are or where the event is being held.
Storing Timezone Information
When storing events in the database, it's prudent to save the event time in UTC and store the intended event timezone in a separate column. This standard practice ensures there's a single point of truth, and converting to local times becomes scalable as your app grows.
In Laravel's migrations, we can define both:
Schema::create('events', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->timestamp('start_time_utc');
$table->string('timezone');
$table->timestamps();
});
When inserting into the database, ensure that the event's starting time is in UTC:
use Illuminate\Support\Facades\DB;
use Carbon\Carbon;
DB::table('events')->insert([
'name' => 'Global Laravel Expo',
'start_time_utc' => Carbon::now('UTC'),
'timezone' => 'Europe/Paris'
]);
Integrating with Luxon.js on the Frontend
Managing timezones isn't just a backend affair; the frontend plays a crucial role and must show times correctly to users. Luxon.js is a superb JavaScript library that pairs nicely with Carbon's backend prowess. Say we fetch our event's UTC time and timezone from Laravel's API. We can now convert and display it to the user's local time using Luxon:
import { DateTime } from 'luxon';
let event = { start_time_utc: '2023-10-30T19:00Z', timezone: 'Europe/Paris' };
let localTime = DateTime
.fromISO(event.start_time_utc, { zone: 'utc' })
.setZone(event.timezone);
console.log(localTime.toString()); // Outputs the event time in 'Europe/Paris' timezone
Ensuring Time Integrity Across Database Types
Laravel's Eloquent ORM is a chameleon, adapting to various database types. Whether you're using MySQL, PostgreSQL, or SQLite, storing timestamps in UTC is a breeze. Laravel takes care of the dirty work, allowing you to focus on what matters—your application's logic. When defining an Eloquent model, you might have:
class Event extends Model
{
protected $casts = [
'start_time_utc' => 'datetime:Y-m-d H:i:s',
];
public function getLocalStartTimeAttribute()
{
return $this->start_time_utc->timezone($this->timezone);
}
}
This cast ensures that whenever start_time_utc is accessed, it's done so as a Carbon instance, ripe for timezone transformation.
Conclusion: Mastering Timezone Management in Laravel
Choosing Laravel for our hypothetical event app has proven sagacious. It gives us the tools, such as Carbon
and now()
, and the functionality, like database-agnostic timezone storage, that make working with timezones not just possible but pleasant.
As developers, we sometimes think of time as an immutable force, but in coding, we have the power to bend it to our will—to make it serve our users, wherever they may be. With Laravel's timeless (pun intended) helpers and the Carbon package, we have the sophistication to handle time with the grace of a world-class event coordinator—ensuring that no matter where on Earth our users may be, the show will indeed go on, right on schedule.
Keep on coding, and rest easy knowing that in Laravel, you're in good times.
Comments (0)
What are your thoughts on "Navigating Timezones in Laravel: Storing and Managing Time with Precision"?
You need to create an account to comment on this post.
Related articles
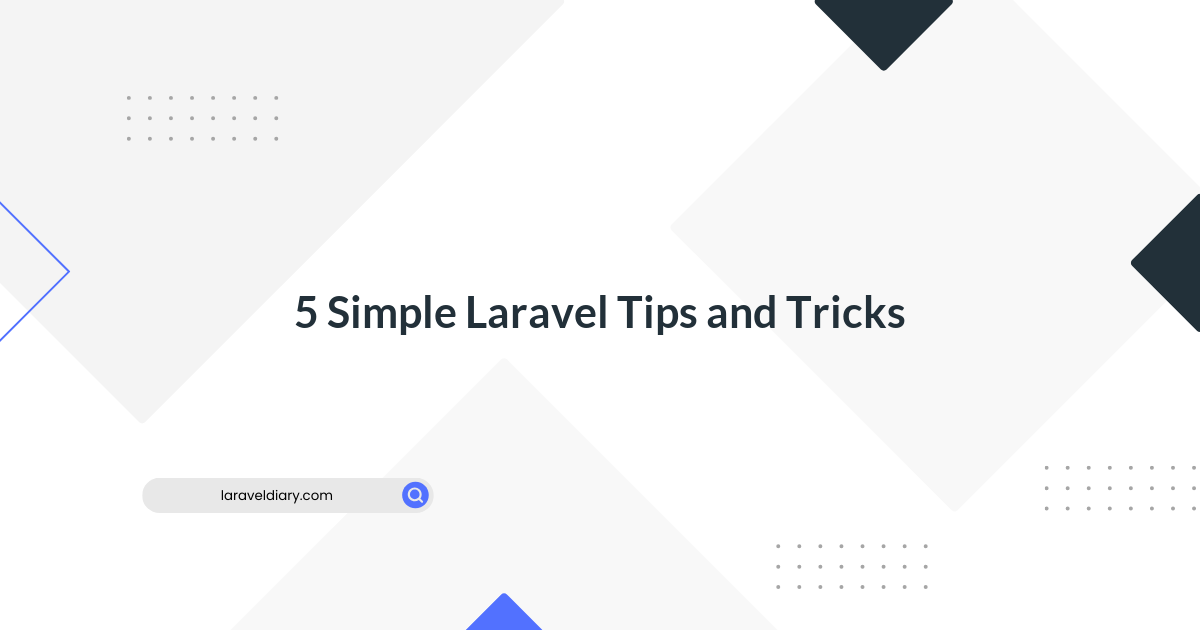