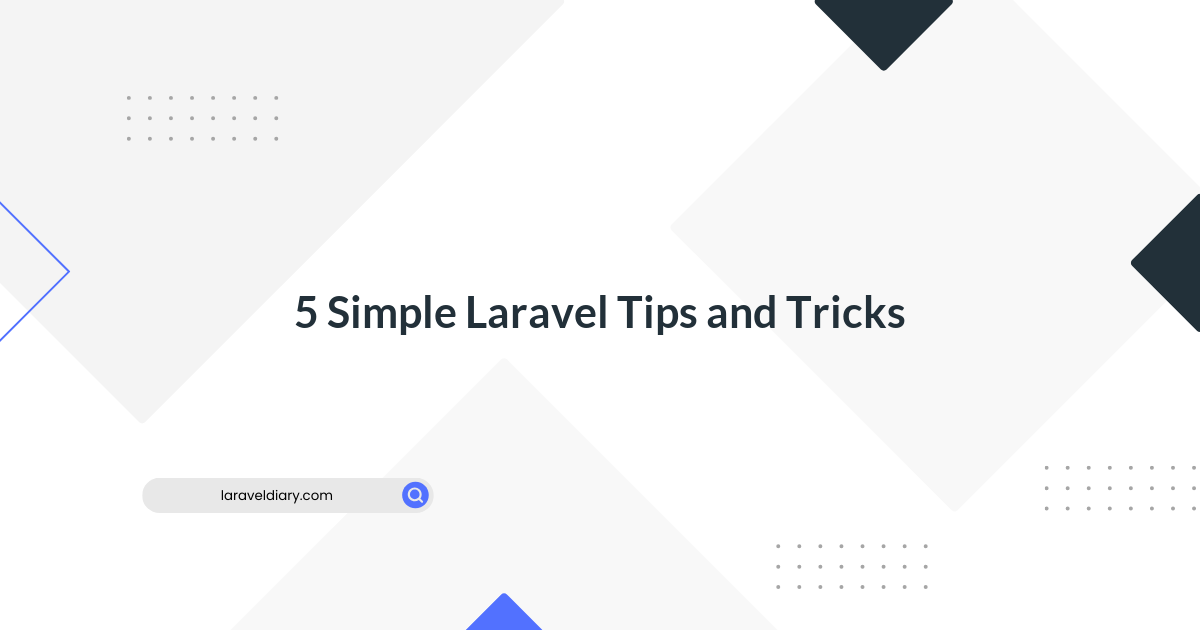
Harnessing Laravel's String Functions for Streamlined App Development
Table of Contents
- The Basics of String Manipulation in Laravel
- Intermediate String Handling Functions
- In Conclusion: Why Laravel String Functions are a Game-Changer
Developing a Content Management System (CMS) with Laravel is akin to painting on a canvas that stretches as far as the eye can see — it's liberating, full of potential, and the results can be nothing short of a masterpiece. Central to this artistic web development process are the string functions that Laravel provides, turning each line of code into a stroke of genius. As a developer who has been through the trenches of CMS app development, I'm here to guide you through the myriad of string functions in Laravel, each with an example to demonstrate their might.
The Basics of String Manipulation in Laravel
Laravel has revolutionized PHP string manipulation, offering a more readable and maintainable way to handle strings with its Str and Stringable classes. Here are some of the most foundational yet indispensable string functions Laravel offers.
Lowercasing and Uppercasing
Simplifying string formatting, Str::lower()
and Str::upper()
are the backbone of consistent text display:
use Illuminate\Support\Str;
echo Str::lower('Make me lower!'); // "make me lower!"
echo Str::upper('raise me up!'); // "RAISE ME UP!"
Perfect for ensuring uniform case usage in your CMS, whether for user-generated content or system messages.
The Title and Slug Transformation
The Str::title()
method capitalizes the first letter of each word, super useful for titling articles in a CMS:
echo Str::title('make this a title'); // "Make This A Title"
And when creating SEO-friendly URLs, Str::slug()
turns a string into a URL-suitable slug:
echo Str::slug('Laravel String Functions!'); // "laravel-string-functions"
CMS developers, rejoice! These functions make managing article titles and URLs a walk in the park.
Trimming Text
CMS content often requires trimming to fit design limitations. The Str::limit()
function does this gracefully without hacking up words:
echo Str::limit('The quick brown fox jumps over the lazy dog', 20); // "The quick brown fox..."
It ensures that your excerpts and summaries stay neat and readable.
Intermediate String Handling Functions
Beyond the basics, Laravel ensures that even complex string manipulations are handled with elegance.
String Replacement and Padding
The Str::replaceFirst()
and Str::replaceLast()
methods allow for surgical replacements:
echo Str::replaceFirst('the', 'a', 'the quick brown fox'); // "a quick brown fox" echo Str::replaceLast('the', 'a', 'the quick brown fox'); // "the quick brown a fox"
This precision is ideal for CMS systems that handle large volumes of text needing specific alterations.
Padding is often overlooked, but with Str::padLeft()
and Str::padRight()
, it couldn't be simpler:
echo Str::padLeft('Laravel', 10, '-'); // "---Laravel"
echo Str::padRight('Laravel', 10, '-'); // "Laravel---"
Ensuring visual alignment in your CMS layout or in coding serialized data is a breeze with these functions.
Working with Substrings
Extracting parts of strings is frequent in CMS work, and the Str::substr()
function is the tool for the job:
echo Str::substr('Laravel Framework', 8); // "Framework"
Advanced Case Conversion
Beyond simple capitalization, Laravel handles kebab-case, snake_case, and camelCase conversions with Str::kebab()
, Str::snake()
, and Str::camel()
respectively:
echo Str::kebab('laravelPaaS'); // "laravel-paas"
echo Str::snake('laravelPaas'); // "laravel_paas"
echo Str::camel('laravel_paas'); // "laravelPaas"
These functions are particularly useful when transitioning between different naming conventions that are a part of CMS development, like URL routing and JavaScript variable naming.
Composing Powerful String Operations
Laravel shines when you chain these functions together to produce powerful one-liners:
use Illuminate\Support\Str;
$headline = Str::of('composing POWERFUL String Operations in Laravel')
->trim()
->lower()
->ucwords()
->replace(' ', '-')
->kebab();
echo $headline; // "composing-powerful-string-operations-in-laravel"
Chaining makes your string manipulation code both eloquent and efficient, reducing clutter and improving readability.
In Conclusion: Why Laravel String Functions are a Game-Changer
Every CMS developer knows that string manipulation is the bread and butter of content management. Laravel's string functions transform what used to be a cumbersome aspect of development into a streamlined, pleasurable process. These functions allow you to write cleaner, more expressive, and more maintainable code that's both easy to read and powerful in execution.
Whether you're building a blogging platform, a news portal, or any sort of CMS, harnessing the power of Laravel's string functions will undoubtedly elevate your development process. The examples provided are just a sampling of what's possible with Laravel's string capabilities — a playground of potential for you to explore and integrate into your app development toolkit.
As we continue to venture deeper into web development, tools like these are not just convenient but essential for crafting applications that stand the test of time and scale. Laravel's string functions are one of many reasons why it remains a beloved framework within the PHP ecosystem. It's clear that when it comes to Laravel, we're not just coding — we're creating art. And with tools like these at our disposal, every CMS we build has the potential to be a masterpiece.
Stay tuned for more deep-dives into Laravel's features, and happy coding!
Comments (0)
What are your thoughts on "Harnessing Laravel's String Functions for Streamlined App Development"?
You need to create an account to comment on this post.
Related articles
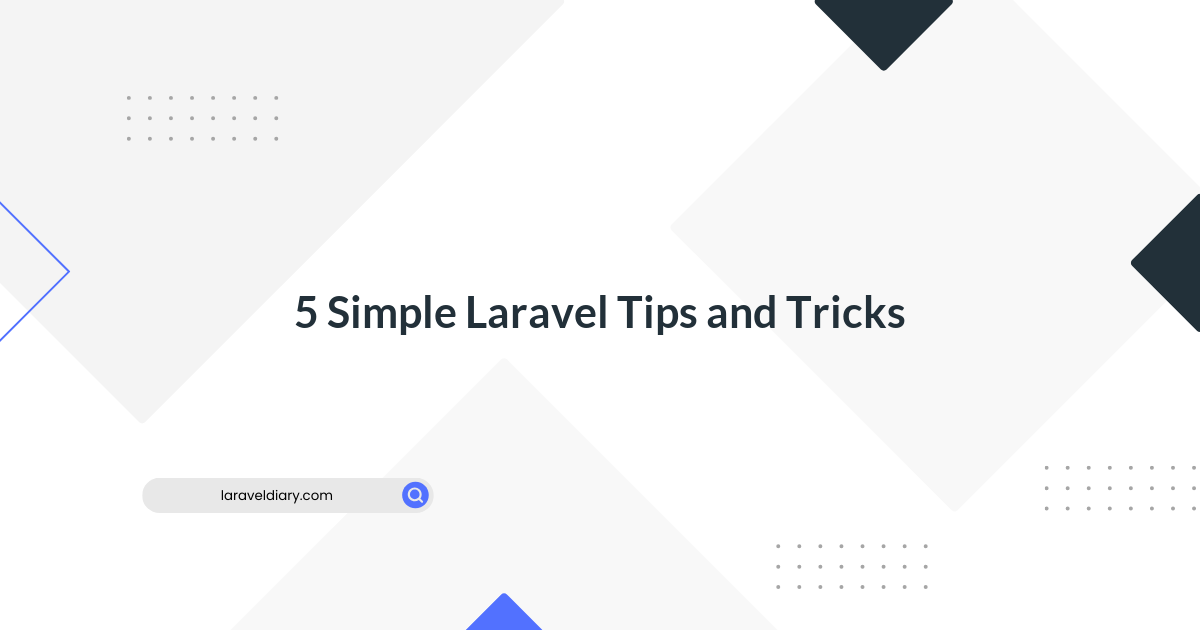