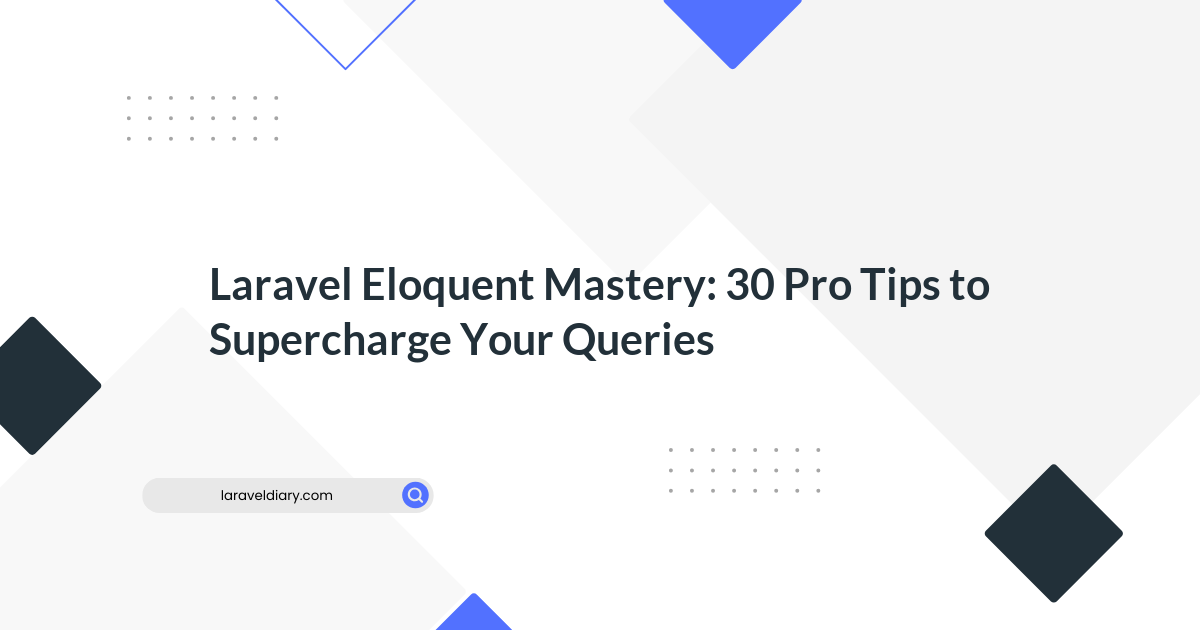
Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries (Series-3)
Table of Contents
- Scenario 11: Selective Relationship Loading with Constraints
- Scenario 12: Using whereColumn for Comparing Columns
- Scenario 13: Scope Query to Current Model Instance
- Scenario 14: Efficient Pivot Table Interaction with wherePivot
- Scenario 15: Prudent Use of latest and oldest
Continuing with the exploration of Laravel Eloquent's advanced tricks, let's walk through another five scenarios that can further enhance the efficiency and robustness of your database queries.
Scenario 11: Selective Relationship Loading with Constraints
Good Practice: Load relations conditionally with constraints to reduce the dataset.
// Load users with their recent active posts
$users = App\\Models\\User::with(['posts' => function ($query) {
$query->where('created_at', '>', now()->subDays(30))
->where('status', 'active');
}])->get();
Bad Practice: Loading all related data regardless of the need.
// Retrieves all posts for each user
$users = App\\Models\\User::with('posts')->get();
whereColumn
for Comparing Columns
Scenario 12: Using Good Practice: Use whereColumn
for intuitive comparisons between two columns.
// Retrieve orders where the shipped date is later than the ordered date
$orders = App\\Models\\Order::whereColumn('shipped_date', '>', 'ordered_date')->get();
Bad Practice: Manual PHP comparisons that can lead to errors and inefficient queries.
$orders = App\\Models\\Order::all()->filter(function ($order) {
return strtotime($order->shipped_date) > strtotime($order->ordered_date);
});
Scenario 13: Scope Query to Current Model Instance
Good Practice: Define local scopes for reusability and maintainability.
class Post extends Model
{
// Define a local scope for published posts
public function scopePublished($query)
{
return $query->where('status', 'published');
}
}
$publishedPosts = App\\Models\\Post::published()->get();
Bad Practice: Repeating query logic throughout the application.
$publishedPosts = App\\Models\\Post::where('status', 'published')->get();
wherePivot
Scenario 14: Efficient Pivot Table Interaction with Good Practice: Use wherePivot
for efficient querying on pivot table fields.
// Retrieve roles of the user where pivot column 'expires_at' is in the future
$userRoles = $user->roles()->wherePivot('expires_at', '>', now())->get();
Bad Practice: Filtering through pivot fields manually.
$userRoles = $user->roles->filter(function ($role) {
return $role->pivot->expires_at > now();
});
latest
and oldest
Scenario 15: Prudent Use of Good Practice: Use latest
and oldest
for a quick order by the creation date.
// Get the latest users by creation date
$latestUsers = App\\Models\\User::latest()->take(10)->get();
Bad Practice: Manually specifying orderBy
for default timestamps.
// More verbose and error-prone
$latestUsers = App\\Models\\User::orderBy('created_at', 'desc')->take(10)->get();
Utilizing these scenarios in your daily development practice with Eloquent ORM will ensure you write more eloquent—pun intended—efficient, and maintainable Laravel code. Always keep looking for ways to refine your queries and embrace Eloquent's rich feature set to its full potential.
Stay tuned as we continue our deep dive into Eloquent's less-traveled paths and reveal more secrets to mastering this ORM.
If you have missed our earlier series you can
Comments (0)
What are your thoughts on "Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries (Series-3)"?
You need to create an account to comment on this post.
Related articles
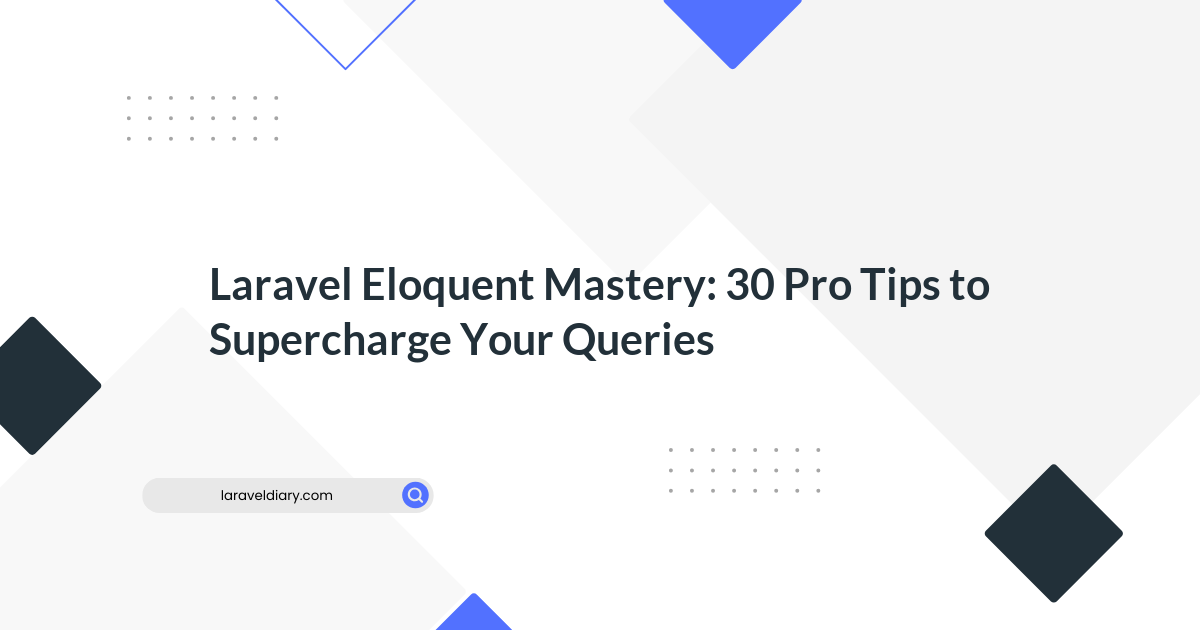