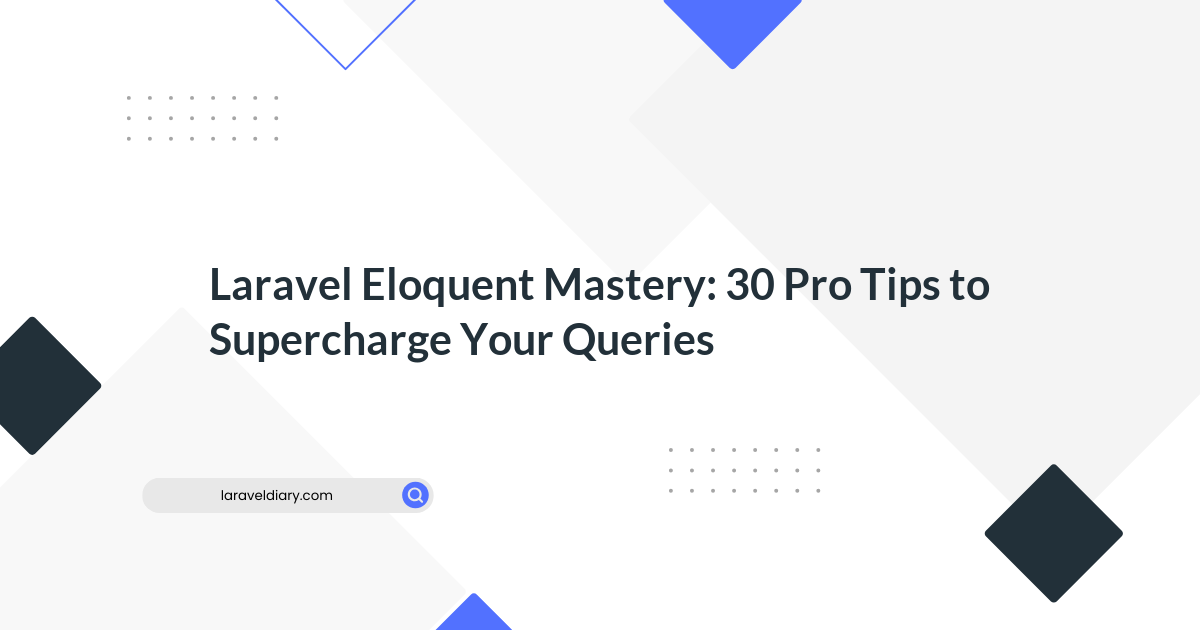
Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries (Series-2)
Table of Contents
- Scenario 6: Efficient Relationship Counts
- Scenario 7: Batch Updates
- Scenario 8: Chunking Results for Memory Efficiency
- Scenario 9: Avoiding Redundant Relationship Queries
- Scenario 10: Cautious Attribute Casting
Scenario 6: Efficient Relationship Counts
Good Practice: Use withCount
for an efficient way to count related models without loading them.
// Get users along with the count of their posts
$users = App\\Models\\User::withCount('posts')->get();
// You can access the count via the {relation}_count attribute
foreach ($users as $user) {
echo $user->posts_count;
}
Bad Practice: Loading the entire relationship only to count it.
$users = App\\Models\\User::with('posts')->get();
foreach ($users as $user) {
echo $user->posts->count();
}
Scenario 7: Batch Updates
Good Practice: Use Eloquent's update
method when performing batch updates.
// Update all posts marked as 'draft' to 'published'
App\\Models\\Post::where('status', 'draft')->update(['status' => 'published']);
Bad Practice: Looping through models to update them individually.
$posts = App\\Models\\Post::where('status', 'draft')->get();
foreach ($posts as $post) {
$post->status = 'published';
$post->save();
}
Scenario 8: Chunking Results for Memory Efficiency
Good Practice: Use chunk
or lazy
to handle large datasets efficiently.
// Process large datasets using chunk to manage memory consumption
App\\Models\\User::where('active', true)->chunk(100, function ($users) {
// Perform actions on chunks of 100 users at a time
});
Bad Practice: Loading entire datasets into memory.
$users = App\\Models\\User::where('active', true)->get(); // Memory-intensive on large datasets
foreach ($users as $user) {
// ...
}
Scenario 9: Avoiding Redundant Relationship Queries
Good Practice: Use the onceWith
method to load a relationship only once.
$users = App\\Models\\User::all();
$users->each(function ($user) {
// The 'profile' relation is loaded only once then reused
$profile = $user->onceWith('profile')->profile;
});
Bad Practice: Loading the same relationship multiple times.
$users = App\\Models\\User::all();
$users->each(function ($user) {
// The 'profile' relationship is loaded for each iteration
$profile = $user->profile;
});
Scenario 10: Cautious Attribute Casting
Good Practice: Cast attributes to the correct type to ensure proper data handling.
class Order extends Model
{
protected $casts = [
'is_processed' => 'boolean',
'order_date' => 'datetime',
];
}
Bad Practice: Ignoring attribute casting, which can lead to unexpected behavior.
class Order extends Model
{
// Lack of casting may result in 'is_processed' being treated as a string
}
Using these advanced Eloquent querying tricks, you can write more efficient and reliable Laravel applications. Observing the good practices demonstrated in the scenarios can significantly improve both the performance of your app and your codebase's maintainability.
Stay tuned for further deep-dives into Eloquent's powerful features and make the most out of your Laravel experience.
If you have missed our earlier series you can [click here](You can continue to read series 2 by clicking here and read it.
Comments (0)
What are your thoughts on "Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries (Series-2)"?
You need to create an account to comment on this post.
Related articles
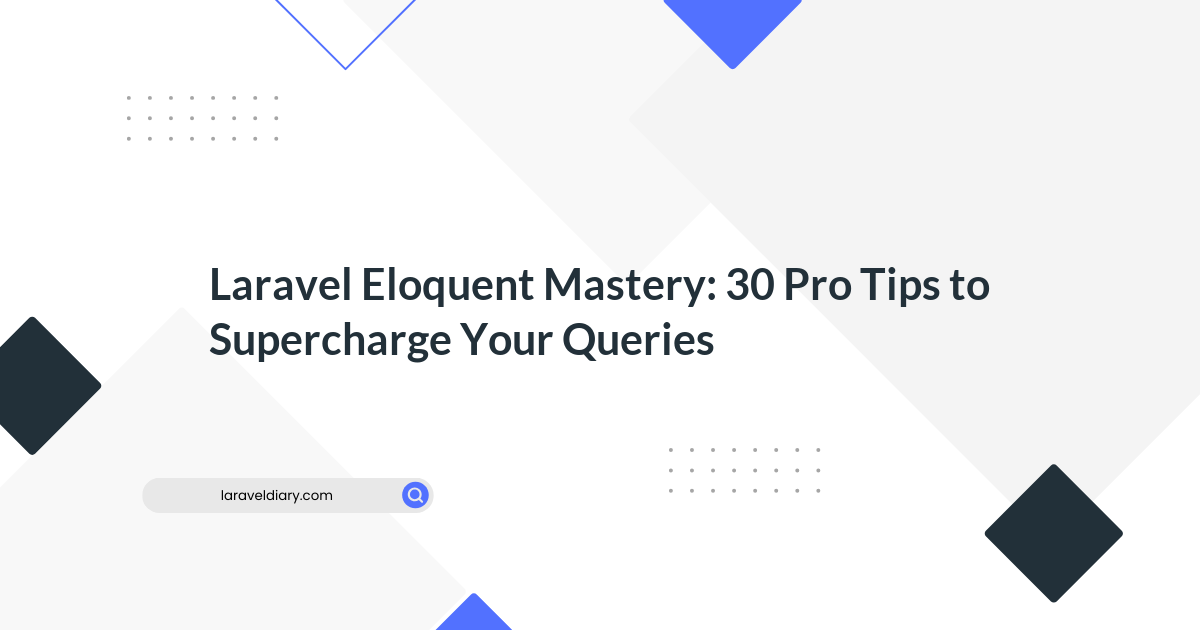