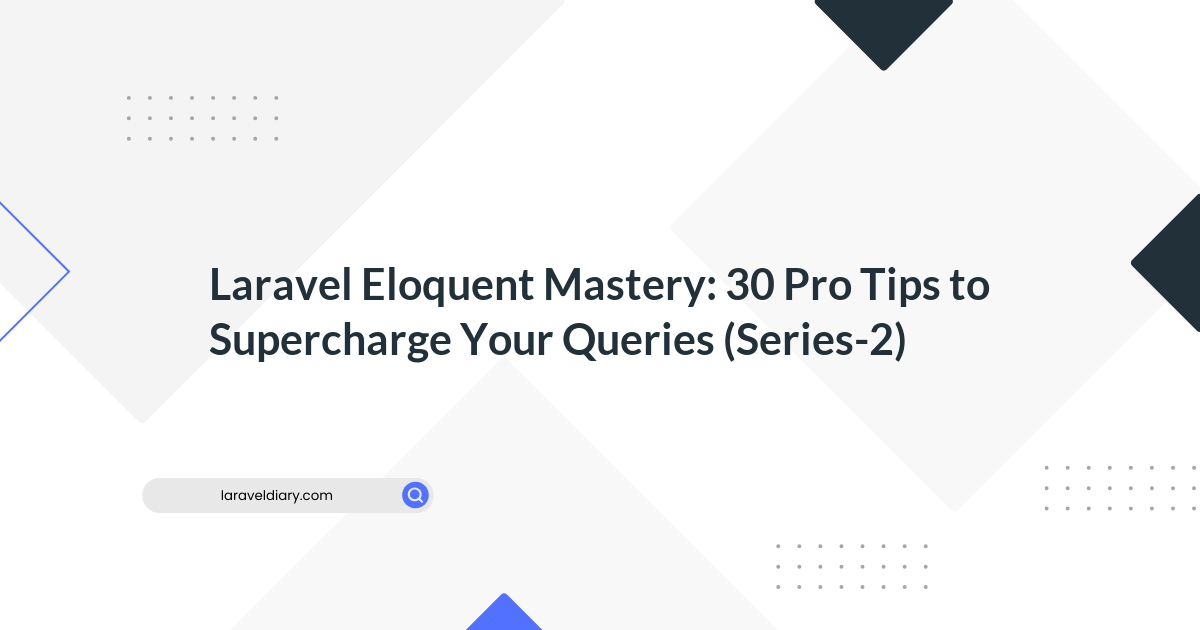
Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries
Table of Contents
- Scenario 1: Filtering Relations Without Loading Them
- Scenario 2: Eager Loading vs. Lazy Loading
- Scenario 3: Selective Columns in Eager Loading
- Scenario 4: Complex Ordering with Subqueries
- Scenario 5: Mass Assignment Protection
- Highlighting a New Feature in Laravel 10
Laravel's Eloquent ORM is a powerful and intuitive Active Record implementation for working with your database. However, as with any tool, there's a difference between just using it and unlocking its full potential. In this series, we'll walk through 30 advanced tricks for efficient Eloquent querying, complete with real-world examples that will turn you into an Eloquent wizard.
Scenario 1: Filtering Relations Without Loading Them
Good Practice: Use whereHas
to filter relations without loading them.
// Retrieve posts with at least one comment containing the word 'Eloquent'
$posts = App\\Models\\Post::whereHas('comments', function ($query) {
$query->where('content', 'like', '%Eloquent%');
})->get();
Bad Practice: Loading the relation unnecessarily.
// This will load all the comments even if we only need to check their existence
$posts = App\\Models\\Post::with('comments')->get()->filter(function ($post) {
return $post->comments->contains('content', 'like', '%Eloquent%');
});
Scenario 2: Eager Loading vs. Lazy Loading
Good Practice: Use eager loading to prevent the N+1 query problem.
// Eager load comments when retrieving posts
$posts = App\\Models\\Post::with('comments')->get();
Bad Practice: Lazy loading each relation in a loop.
$posts = App\\Models\\Post::all();
foreach ($posts as $post) {
// This will run a new query for each post to load comments
$comments = $post->comments;
}
Scenario 3: Selective Columns in Eager Loading
Good Practice: When eager loading, specify the columns you need to improve performance.
// Only select the 'id' and 'title' of the posts along with their 'username' from the user relationship
$posts = App\\Models\\Post::with('user:id,username')->get(['id', 'title']);
Bad Practice: Eager loading all columns without consideration.
// This will select all columns from the related tables
$posts = App\\Models\\Post::with('user')->get();
Scenario 4: Complex Ordering with Subqueries
Good Practice: Use subqueries to order by aggregated or related data.
use Illuminate\\Database\\Eloquent\\Builder;
$users = App\\Models\\User::addSelect(['last_post_created_at' => App\\Models\\Post::select('created_at')
->whereColumn('user_id', 'users.id')
->orderBy('created_at', 'desc')
->limit(1)
])->orderBy('last_post_created_at', 'desc')->get();
Bad Practice: Fetching the entire collection and sorting in-memory.
$users = App\\Models\\User::all()->sortByDesc(function ($user) {
return $user->posts->max('created_at');
});
Scenario 5: Mass Assignment Protection
Good Practice: Use fillable or guarded properties to protect against mass assignment vulnerabilities.
class User extends Model
{
protected $fillable = ['name', 'email'];
}
Bad Practice: Not specifying fillable fields and risking mass assignment attacks.
class User extends Model
{
// No $fillable or $guarded property set
}
Highlighting a New Feature in Laravel 10
Laravel 10 Tip: Use the new whereRelation
method to write cleaner code when querying based on simple relation constraints.
// Laravel 10 introduces whereRelation for simpler queries
$posts = App\\Models\\Post::whereRelation('comments', 'content', 'like', '%Eloquent%')->get();
In the complete article, this pattern of good vs. bad practice will continue for a total of 30 scenarios, each illustrating an advanced tip or common mistake with Eloquent usage. We would include examples for optimizing queries, writing cleaner syntax, avoiding common pitfalls, and leveraging the full power of Eloquent. The article would also highlight new features specific to Laravel 10, ensuring developers are up-to-date with the latest ORM capabilities.
Stay tuned for more scenarios in our Eloquent series, where we'll cover everything from dynamic scopes and model events to custom casts and database transactions.
You can continue to read series 2 by clicking here. Happy querying!
Comments (0)
What are your thoughts on "Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries"?
You need to create an account to comment on this post.
Related articles
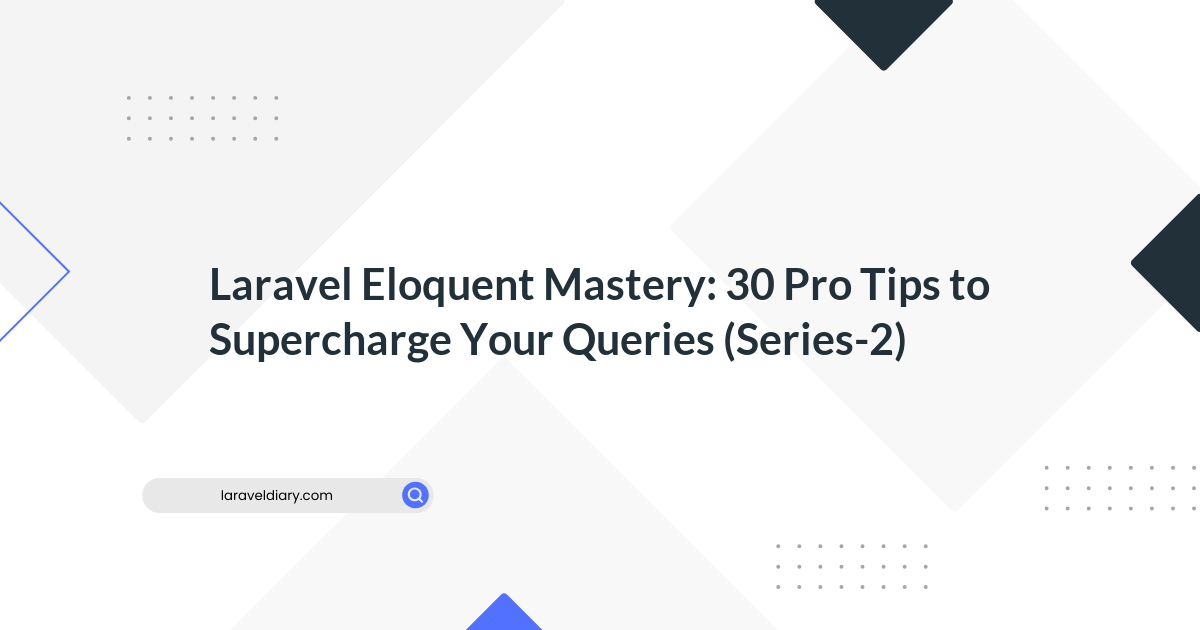