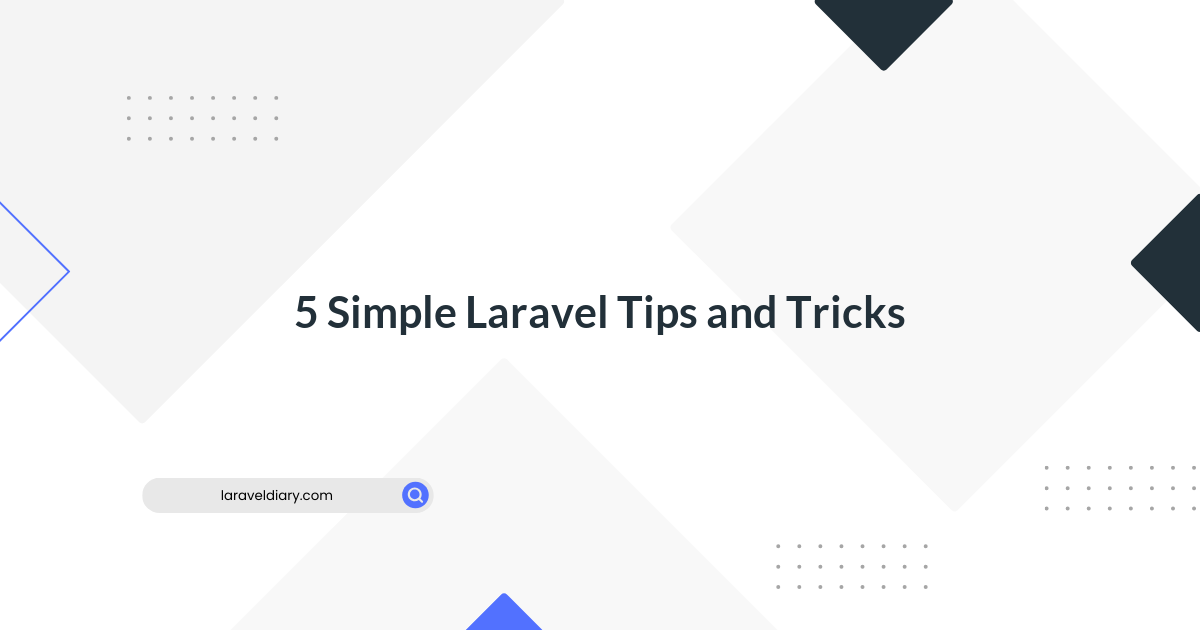
Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries (Series-5)
Table of Contents
- Scenario 21: Utilizing Subquery Selects
- Scenario 22: The Power of withSum, withAvg, and Related Methods
- Scenario 23: Use of findMany for Multiple Models
- Scenario 24: The Usefulness of sole Method
- Scenario 25: Leveraging lockForUpdate
Continuing with our exploration of advanced Eloquent techniques, let's examine five additional scenarios to ensure your database queries are as efficient as possible.
Scenario 21: Utilizing Subquery Selects
Good Practice: Use subquery selects to include information from related tables in your initial query results, avoiding the N+1 problem.
// Add the latest post title to each user result
$users = App\\Models\\User::addSelect(['latest_post_title' => App\\Models\\Post::select('title')
->whereColumn('user_id', 'users.id')
->latest()
->limit(1)
])->get();
Bad Practice: Attaching related data via a loop; this leads to unnecessary queries.
$users = App\\Models\\User::all()->each(function ($user) {
$user->latest_post_title = $user->posts()->latest()->first()->title;
});
withSum
, withAvg
, and Related Methods
Scenario 22: The Power of Good Practice: Optimize summary calculations on relationships using Eloquent's withSum
, withAvg
, and other similar methods.
// Get users with the sum of their order amounts
$users = App\\Models\\User::withSum('orders', 'amount')->get();
Bad Practice: Performing manual calculations after loading relationships.
$users = App\\Models\\User::with('orders')->get()->each(function ($user) {
$user->orders_sum = $user->orders->sum('amount');
});
findMany
for Multiple Models
Scenario 23: Use of Good Practice: Efficient retrieval of many models by an array of primary keys using findMany
.
// Get multiple posts by an array of IDs
$postIds = [1, 2, 3];
$posts = App\\Models\\Post::findMany($postIds);
Bad Practice: Looping through IDs to retrieve models one at a time.
$postIds = [1, 2, 3];
$posts = collect($postIds)->map(function ($id) {
return App\\Models\\Post::find($id);
});
sole
Method
Scenario 24: The Usefulness of Good Practice: Using sole
method to retrieve a single record that matches the query constraints and fail if there are no or multiple records.
// Retrieve a single user by email or fail if it does not exist or multiple exist
$user = App\\Models\\User::where('email', '[email protected]')->sole();
Bad Practice: Manually verifying the number of records to find a single expected entry.
$users = App\\Models\\User::where('email', '[email protected]')->get();
if ($users->count() !== 1) {
throw new Exception('Expected only one user.');
}
$user = $users->first();
lockForUpdate
Scenario 25: Leveraging Good Practice: Use lockForUpdate
to prevent the selected rows from being modified or from selecting by other shared lock until the transaction is complete when dealing with concurrent database access.
DB::transaction(function () {
$affectedRows = App\\Models\\User::where('votes', '>', 100)->lockForUpdate()->get();
// Perform operation on the affected rows
});
Bad Practice: Not using proper locking mechanisms, which can lead to race conditions and data corruption.
$affectedRows = App\\Models\\User::where('votes', '>', 100)->get();
// If another process modifies the data here, it could cause issues
// Perform operation on the affected rows
Incorporating these practices within your Laravel projects can vastly improve your Eloquent ORM fluency and ensure that your applications run optimally. It emphasizes the importance of using the right tool for the job — in this case, Eloquent methods that have been crafted to solve specific challenges when dealing with database records. By mastering these scenarios, your database interactions will become more streamlined and reliable. Keep an eye out for our next batch of scenarios to round out your Eloquent expertise!
Stay tuned as we continue our deep dive into Eloquent's less-traveled paths and reveal more secrets to mastering this ORM.
If you have missed our earlier series you can
Comments (0)
What are your thoughts on "Laravel Eloquent Mastery: 30 Pro Tips to Supercharge Your Queries (Series-5)"?
You need to create an account to comment on this post.
Related articles
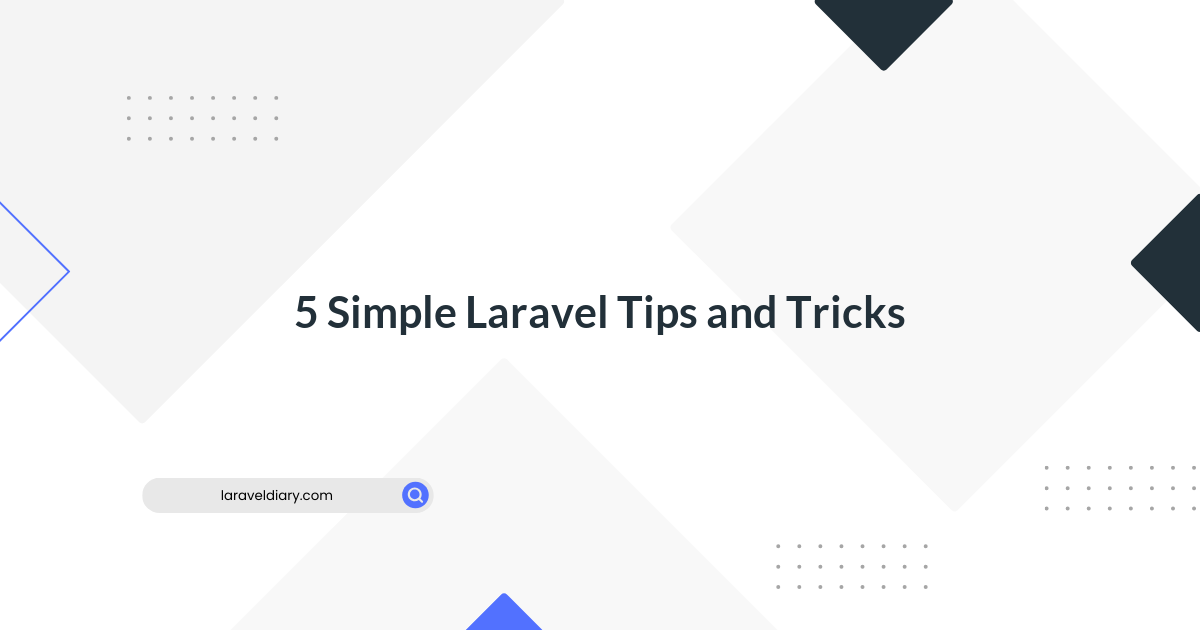