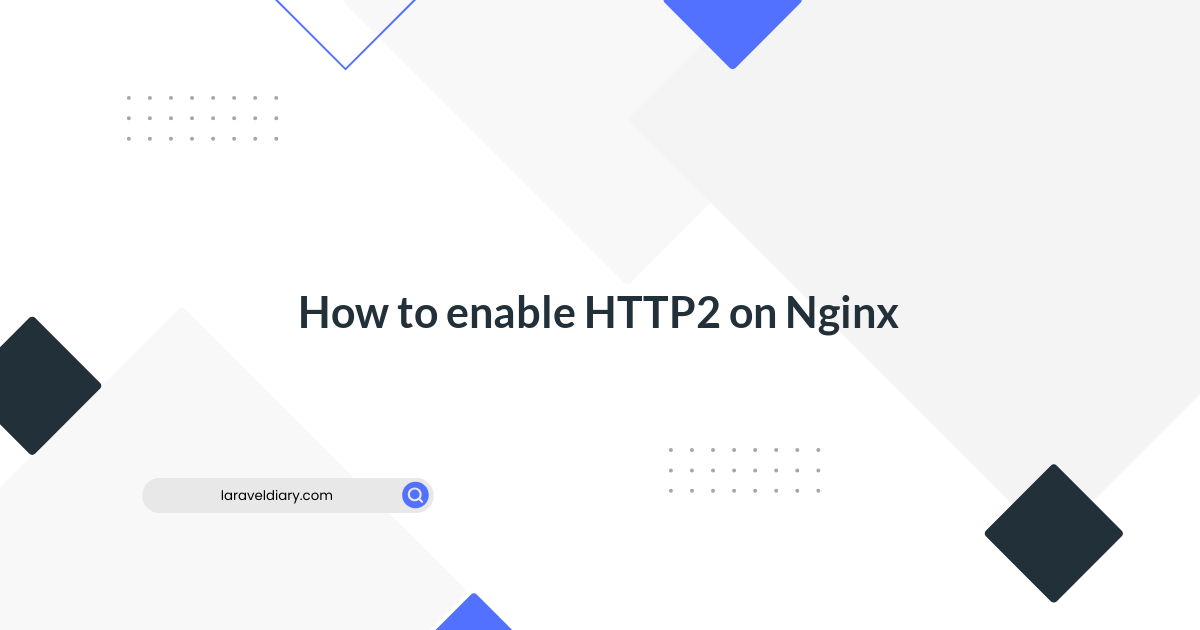
Optimizing Laravel on AWS EC2: A Guide to Activating HTTP/2 & HTTP/3 Using Nginx
Table of Contents
- Step 1: Setting Up the Stage - AWS EC2
- Step 2: Meet Nginx - The Heart of Performance
- Step 3: PHP.ini – Turning the Knobs for Performance
- Step 4: Securing Your Setup
- Step 5: Serve Laravel with Nginx
Hello, fellow developers! Today's the day we supercharge our Laravel application by setting it up with Nginx on AWS EC2. We're not just going for the industry standard here – we're pushing the envelope by enabling HTTP/2 and even HTTP/3, ensuring our Laravel applications are not just safe and sound, but also lightning-fast!
Step 1: Setting Up the Stage - AWS EC2
First things first, we'll need a robust server. I trust you're already familiar with AWS's EC2, so let's get that instance running. For our Laravel app, let's choose Ubuntu, thanks to its stellar performance and security updates. Spin up an EC2 instance using the Ubuntu Server 20.04 LTS, which provides just the right environment for Nginx and PHP.
Step 2: Meet Nginx - The Heart of Performance
Nginx is our web server of choice. Why so? Because it's fast, reliable, and now with HTTP/2 and HTTP/3 support, it's like putting the pedal to the metal.
Install Nginx with HTTP/2 Support
sudo apt update
sudo apt install nginx
Editing Nginx to enable HTTP/2 is straightforward:
# In /etc/nginx/nginx.conf
server {
listen 443 ssl http2;
server_name example.com;
ssl_certificate /etc/nginx/ssl/example.com.crt;
ssl_certificate_key /etc/nginx/ssl/example.com.key;
# Additional SSL settings...
# ...
}
HTTP/2 is now ready and will serve your content more efficiently than ever!
Upgrading to HTTP/3 – A Leap into the Future
HTTP/3 support requires a bit of extra work as Nginx doesn't support it natively yet (as of my last update). We'll need to compile Nginx from source with a library like quiche that supports HTTP/3.
sudo apt install git build-essential cmake go libpcre2-dev zlib1g-dev libev-dev libssl-dev -y
git clone --recursive https://github.com/cloudflare/quiche
cd quiche/extras/nginx/
QUICHE_BSSL_PATH=$HOME/quiche/deps/boringssl QUICHE_INCLUDE_PATH=$HOME/quiche/include QUICHE_LIB_PATH=$HOME/quiche/target/release
./configure --prefix=/etc/nginx --with-http_v3_module --with-openssl=$QUICHE_BSSL_PATH --with-openssl-opt=enable-tls1_3
make && sudo make install
After this, update your nginx.conf
to add the HTTP/3 module settings, and you're blazing trails on the superhighway of the web.
Step 3: PHP.ini – Turning the Knobs for Performance
For Laravel to work smoothly with our Nginx setup, we need to tweak our php.ini
. Here are a few strategic changes to make sure PHP-FPM doesn't slack off:
; In /etc/php/7.4/fpm/php.ini
memory_limit = 256M
upload_max_filesize = 50M
post_max_size = 50M
max_execution_time = 300
date.timezone = Your/Timezone
opcache.enable=1
opcache.revalidate_freq=2
opcache.validate_timestamps=1
opcache.max_accelerated_files=7963
opcache.memory_consumption=192
opcache.interned_strings_buffer=16
opcache.fast_shutdown=1
Why these changes? They're all about optimizing execution time, memory allocation, and making sure the Opcache is tuned correctly to give you the performance you crave.
Step 4: Securing Your Setup
Security is paramount, and since we're here, why not shore up our defenses? Here's a basic setting you should apply:
# In /etc/nginx/nginx.conf
server_tokens off;
add_header X-Frame-Options \"SAMEORIGIN\";
add_header X-Content-Type-Options \"nosniff\";
add_header X-XSS-Protection \"1; mode=block\";
ssl_protocols TLSv1.3; # Or TLSv1.2 if you need to maintain backward compatibility
These headers and SSL protocol settings help in mitigating common web vulnerabilities and ensuring the communication is secure.
Step 5: Serve Laravel with Nginx
Finally, configure Nginx to serve your Laravel app. Use PHP-FPM to process PHP requests and make sure Nginx knows where to find the public
directory of your Laravel app.
# In /etc/nginx/sites-available/example.com
server {
listen 80;
server_name example.com;
return 301 https://$server_name$request_uri;
}
server {
listen 443 ssl http2;
server_name example.com;
root /var/www/example.com/public;
index index.php index.html index.htm index.nginx-debian.html;
location / {
try_files $uri $uri/ /index.php?$query_string;
}
location ~ \\.php$ {
include snippets/fastcgi-php.conf;
fastcgi_pass unix:/var/run/php/php7.4-fpm.sock;
}
# Additional settings...
# ...
}
After each modification, make sure to check your configuration and restart Nginx:
sudo nginx -t
sudo systemctl restart nginx
You've just set up your Laravel app on EC2, with HTTP/2 enabled and HTTP/3 ready to go. Your app isn't just running; it's operating at peak efficiency, thanks to Nginx's performance and the fine-tuned PHP setup.
With the right configurations, setting up Laravel with Nginx on AWS EC2, complete with HTTP/2 and HTTP/3, is a hallmark of elite web app performance. So roll up your sleeves, apply these fine-tuned configurations, and watch your Laravel application soar to new heights.
Happy deploying, and may your user experiences be swift and secure!
Comments (0)
What are your thoughts on "Optimizing Laravel on AWS EC2: A Guide to Activating HTTP/2 & HTTP/3 Using Nginx"?
You need to create an account to comment on this post.